문제 1.
차량을 2초간 바라보면 메뉴 UI를 보여줍니다.
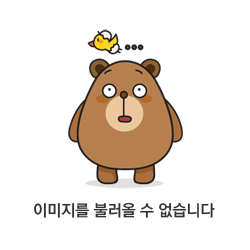
문제 2.
메뉴가 열렸다면 메뉴를 응시 하면 게이지가 비워져야 합니다 (버튼 인터렉션을 위해)
다시 차량을 응시 했다면 메뉴가 열려 있기 때문에 게이지가 채워져 있어야 합니다.
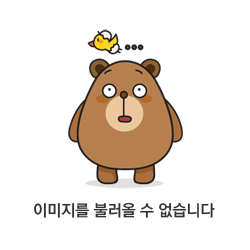
문제 3
예 버튼을 2초간 응시 했을경우 예를 선택 했습니다를 출력 합니다
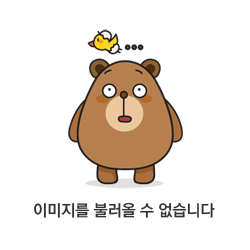
문제 4 번
아니오 버튼을 2초간 응시 했을 경우 아니오를 선택 했습니다를 출력 하고 메뉴를 닫습니다.
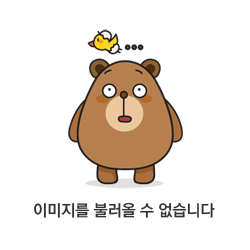
using System.Collections;
using UnityEngine;
using UnityEngine.UI;
public class EyeCast : MonoBehaviour
{
private Transform trans;
private RaycastHit hit;
public float dist = 10f;
public Image gauge;
public Car car;
public Button clickYes;
public Button clickNo;
private int lastLayer = 0;
private string lastButton = "";
float carCount = 0;
float yesButtonCount = 0;
float noButtonCount = 0;
void Start()
{
this.trans = this.GetComponent<Transform>();
car.menuUI.SetActive(false); // 처음엔 menu가 닫힌채로 시작
StartCoroutine(ReleaseButton());
StartCoroutine(GazeButton());
StartCoroutine(ClickButton());
}
IEnumerator GazeButton()
{
while (true)
{
Ray ray = new Ray(this.trans.position, this.trans.forward);
if (Physics.Raycast(ray, out hit, dist, 1 << 7)) // Layer 7 = Car
{
if (lastLayer != 7 && carCount < 2)
{
carCount = 0;
gauge.fillAmount = 0;
}
else if (lastLayer != 7 && carCount >= 2)
{
carCount = 2;
gauge.fillAmount = 1;
}
else if (lastLayer !=7 && carCount >= 2 && !car.menuUI.gameObject.activeSelf) // No 버튼으로 menu가 닫혔을때, 다시 초기화
{
carCount = 0;
gauge.fillAmount = 0;
}
lastLayer = 7;
lastButton = "";
//car.menuUI.SetActive(true); -> Car를 응시 했을 때, 바로 메뉴 오픈
if (carCount < 2)
{
carCount += Time.deltaTime;
gauge.fillAmount = carCount / 2;
if (gauge.fillAmount >= 1)
{
car.menuUI.SetActive(true); // -> Car를 응시 했을 때, 2초 후 메뉴 오픈
}
}
}
yield return null;
}
}
IEnumerator ClickButton() // ButtonYes와 ButtonNo 콜라이더 사이에 공백이 있으면 ResetCountAndGauge 메서드(메뉴 닫힘 및 초기화)가 실행 될 수 있으니 유의
{
while (true)
{
Ray ray = new Ray(this.trans.position, this.trans.forward);
if (Physics.Raycast(ray, out hit, dist, 1 << 6)) //Layer 6 = Button
{
if (hit.collider.gameObject.name == "ButtonYes")
{
lastLayer = 6;
if (lastButton != "ButtonYes" && yesButtonCount < 2)
{
lastButton = "ButtonYes";
yesButtonCount = 0;
gauge.fillAmount = 0;
}
else if (lastButton != "ButtonYes" && yesButtonCount >= 2)
{
lastButton = "ButtonYes";
yesButtonCount = 2;
gauge.fillAmount = 1;
}
if (yesButtonCount < 2)
{
yesButtonCount += Time.deltaTime;
gauge.fillAmount = yesButtonCount / 2;
if (gauge.fillAmount >= 1)
{
Debug.Log("예를 입력하였습니다");
car.uiText.text = "차량 정보 확인"; // UI Text 변경
}
}
}
else if (hit.collider.gameObject.name == "ButtonNo")
{
lastLayer = 6;
if (lastButton != "ButtonNo")
{
lastButton = "ButtonNo";
noButtonCount = 0;
gauge.fillAmount = 0;
}
if (noButtonCount < 2)
{
noButtonCount += Time.deltaTime;
gauge.fillAmount = noButtonCount / 2;
if (gauge.fillAmount >= 1)
{
car.menuUI.SetActive(false);
// Count 및 Text 초기화
car.uiText.text = "차량의 정보를 보시겠습니까?";
carCount = 0;
yesButtonCount = 0;
noButtonCount = 0;
Debug.Log("아니오를 입력하였습니다. 메뉴를 종료합니다.");
}
}
}
}
yield return null;
}
}
IEnumerator ReleaseButton()
{
while (true)
{
Ray ray = new Ray(this.trans.position, this.trans.forward);
if (!Physics.Raycast(ray, out hit, dist))
{
//car.menuUI.SetActive(false); -> Car, 버튼 어느것도 응시하지 않으면 menu 닫음
gauge.fillAmount = 1;
lastLayer = 0;
lastButton = "";
}
yield return null;
}
}
}
EyeCast.cs
using System.Collections;
using System.Collections.Generic;
using UnityEditor;
using UnityEngine;
using UnityEngine.EventSystems;
using UnityEngine.InputSystem.LowLevel;
using UnityEngine.UI;
public class Car : MonoBehaviour
{
private Coroutine coroutine;
public GameObject menuUI;
public Text uiText; // menuUI Text
}
Car.cs
+++
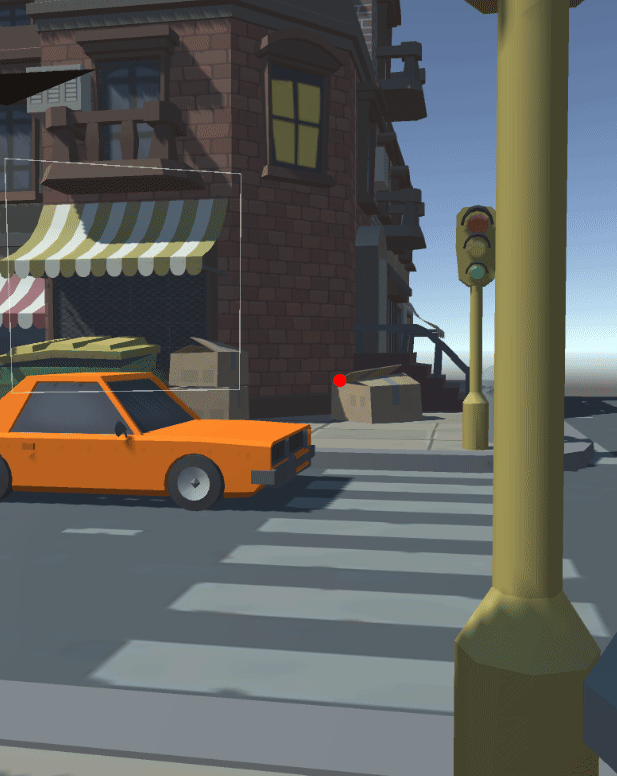
1번
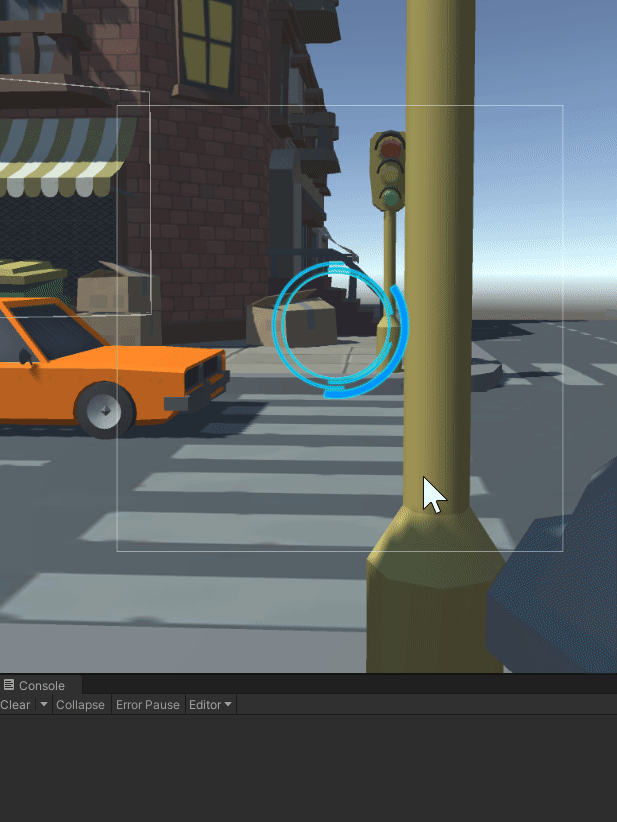
2번
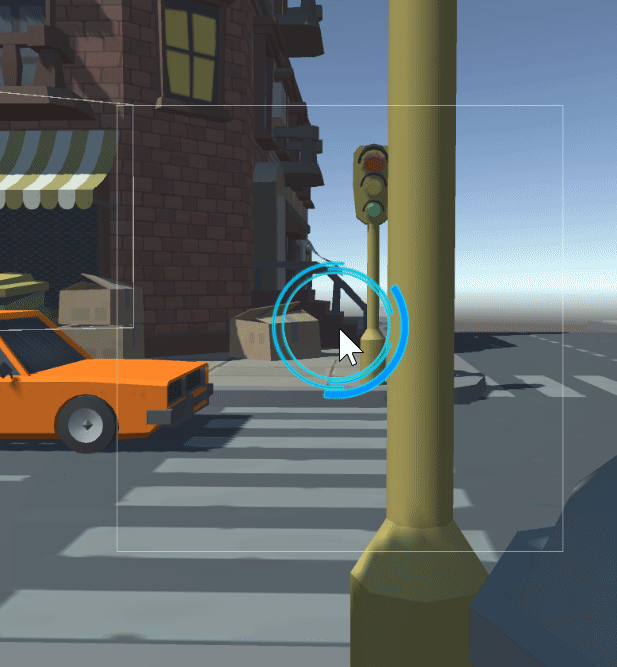
3번
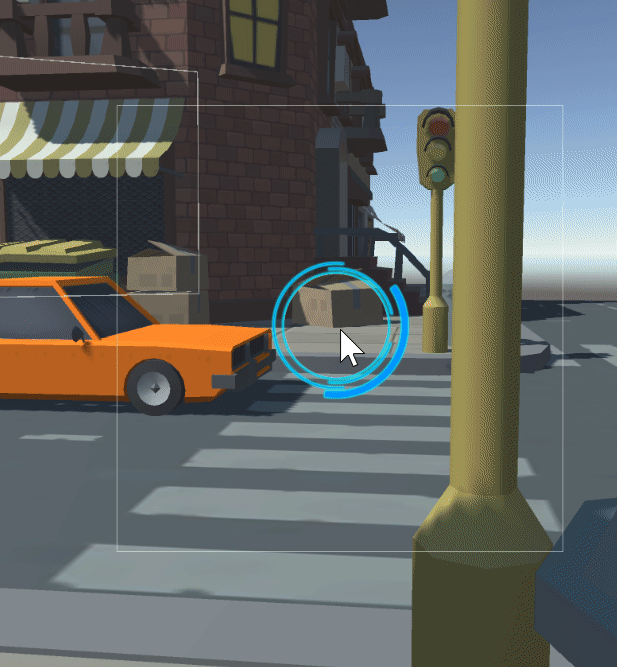
4번
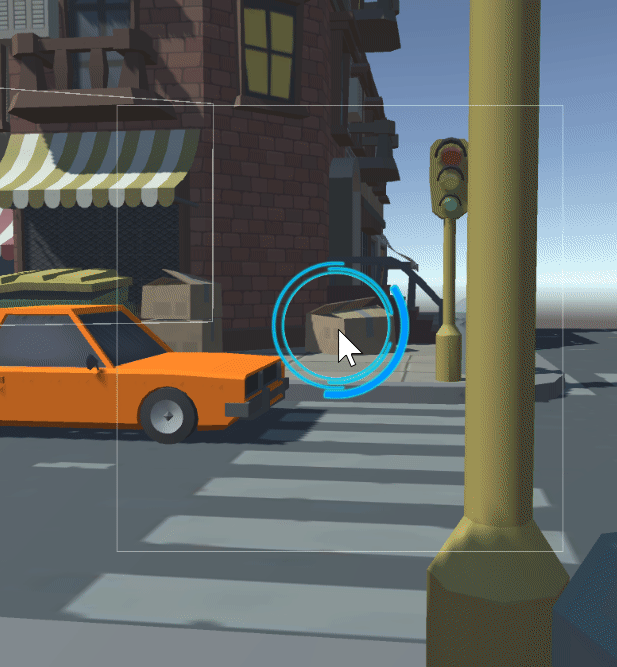
5번
using System.Collections;
using UnityEngine;
using UnityEngine.UI;
public class EyeCast : MonoBehaviour
{
private Transform trans;
private RaycastHit hit;
public float dist = 10f;
public Image gauge;
public Car car;
public Button clickYes;
public Button clickNo;
private int lastLayer = 0;
private string lastButton = "";
float carCount = 0;
float yesButtonCount = 0;
float noButtonCount = 0;
void Start()
{
this.trans = this.GetComponent<Transform>();
car.menuUI.SetActive(false); // 처음엔 menu가 닫힌채로 시작
StartCoroutine(ReleaseButton());
StartCoroutine(GazeButton());
StartCoroutine(ClickButton());
}
IEnumerator GazeButton()
{
while (true)
{
Ray ray = new Ray(this.trans.position, this.trans.forward);
if (Physics.Raycast(ray, out hit, dist, 1 << 7)) // Layer 7 = Car
{
if (lastLayer != 7 && carCount < 2)
{
carCount = 0;
gauge.fillAmount = 0;
}
else if (lastLayer != 7 && carCount >= 2)
{
carCount = 2;
gauge.fillAmount = 1;
}
else if (lastLayer !=7 && carCount >= 2 && !car.menuUI.gameObject.activeSelf) // No 버튼으로 menu가 닫혔을때, 다시 초기화
{
carCount = 0;
gauge.fillAmount = 0;
}
lastLayer = 7;
lastButton = "";
//car.menuUI.SetActive(true); -> Car를 응시 했을 때, 바로 메뉴 오픈
if (carCount < 2)
{
carCount += Time.deltaTime;
gauge.fillAmount = carCount / 2;
if (gauge.fillAmount >= 1)
{
car.menuUI.SetActive(true); // -> Car를 응시 했을 때, 2초 후 메뉴 오픈
//Debug.Log("메뉴를 보여줍니다.");
}
}
}
yield return null;
}
}
IEnumerator ClickButton() // ButtonYes와 ButtonNo 콜라이더 사이에 공백이 있으면 ResetCountAndGauge 메서드(메뉴 닫힘 및 초기화)가 실행 될 수 있으니 유의
{
while (true)
{
Ray ray = new Ray(this.trans.position, this.trans.forward);
if (Physics.Raycast(ray, out hit, dist, 1 << 6)) //Layer 6 = Button
{
if (hit.collider.gameObject.name == "ButtonYes")
{
lastLayer = 6;
if (lastButton != "ButtonYes" && yesButtonCount < 2)
{
lastButton = "ButtonYes";
yesButtonCount = 0;
gauge.fillAmount = 0;
}
else if (lastButton != "ButtonYes" && yesButtonCount >= 2)
{
lastButton = "ButtonYes";
yesButtonCount = 2;
gauge.fillAmount = 1;
}
if (yesButtonCount < 2)
{
yesButtonCount += Time.deltaTime;
gauge.fillAmount = yesButtonCount / 2;
if (gauge.fillAmount >= 1)
{
Debug.Log("예를 입력하였습니다");
car.uiText.text = "예"; // UI Text 변경
}
}
}
else if (hit.collider.gameObject.name == "ButtonNo")
{
lastLayer = 6;
if (lastButton != "ButtonNo")
{
lastButton = "ButtonNo";
noButtonCount = 0;
gauge.fillAmount = 0;
}
if (noButtonCount < 2)
{
noButtonCount += Time.deltaTime;
gauge.fillAmount = noButtonCount / 2;
if (gauge.fillAmount >= 1)
{
car.uiText.text = "아니오"; // UI Text 변경
yield return new WaitForSeconds(1f);
Debug.Log("아니오를 입력하였습니다. 메뉴를 종료합니다.");
car.menuUI.SetActive(false);
// Count 및 Text 초기화
car.uiText.text = "차량의 정보를 보시겠습니까?";
carCount = 0;
yesButtonCount = 0;
noButtonCount = 0;
}
}
}
}
yield return null;
}
}
IEnumerator ReleaseButton()
{
while (true)
{
Ray ray = new Ray(this.trans.position, this.trans.forward);
if (!Physics.Raycast(ray, out hit, dist))
{
//car.menuUI.SetActive(false); //-> Car, 버튼 어느것도 응시하지 않으면 menu 닫음
gauge.fillAmount = 1;
lastLayer = 0;
lastButton = "";
}
yield return null;
}
}
}
반응형
'산대특 > VR 콘텐츠 기초 및 구현' 카테고리의 다른 글
Locomotion (0) | 2024.04.23 |
---|---|
Create a Flat UI (0) | 2024.04.23 |
Create Ray Interactions (0) | 2024.04.23 |
Box Grab Surface (0) | 2024.04.19 |
Event Trigger로 해당 물체를 응시하면 응시 이벤트 처리해보기 (0) | 2024.04.12 |