오늘은 Windows Forms를 이용하여
수학 퀴즈 앱을 만들어 보았다.
참고 공식 문서는
자습서: ‘수학 퀴즈’ Windows Forms 앱 만들기 - Visual Studio (Windows)
수학 퀴즈 애플리케이션에 대한 C# 또는 Visual Basic Windows Forms 프로젝트를 만들고 Visual Studio를 사용하여 양식에 UI 컨트롤을 추가합니다.
learn.microsoft.com
를 참고 했다.
+++
'MathQuiz' 로 프로젝트 생성
속성 - Text 속성을 'Math Quiz'로 변경
속성창에서 form의 크기를 500 x 400으로 설정
FormBorderStyle - Fixed3D로 설정
MaximizeBox - False로 설정
도구상자에서 Label을 생성
Name - timeLabel
AutoSize - False
BorderStyle - FixedSingle
Size - 200x30
Text - "" (빈칸으로 설정)
Font - Size - 15.75
Label 하나 더 생성
마찬가지로
Font - Size - 15.75 설정
Text - Time Left
옆의 Label과 위치 맞추기
Label 하나 더 생성
Text - ?
AutoSize - False
Size - 60x50
Font - Size - 18
TextAlign - MiddleCenter
Location - 50, 75
Name - plusLeftLabel
레이블을 세번 복사 (ctrl + v)
복사된 레이블이 나란히 놓이도록 정렬 및 위치 시키기
두번째 레이블의 Text - +
세번째 레이블의 Name - plusRightLabel
네번째 레이블의 Text - =
도구상자에서 NumericUpDown을 Form에 생성 및 레이블과 나란히 위치 시켜주기
numericUpDown - Name - sum
Size - Width - 100
Font - Size - 18
첫번째 줄의 Label 및 NumericUpDown을 복사
첫번째 Label - Name - minusLeftLabel
두번째 Label - Text - -
('-'로 설정)
세번째 Label - Name - minusRightLabel
NumericUpDown - Name - difference
만들었던 두 줄(Label, NumericUpDown)을 한번 더 복사
[세번째 행]
첫번째 Label - Name - timesLeftLabel
두번째 Label - Text - x
세번째 Label - Name - timesRightLabel
NumericUpDown - Name - product
[네번째 헹]
첫번째 Label - Name - dividedLeftLabel
두번째 Label - Text - ÷
세번째 Label - Name - dividedRightLabel
NumericUpDown - Name - quotient
도구상자에서 Button 생성
Name - startBtn
Text - Start the quiz
Font - Size - 14
AutoSize - True
TabIndex - 0
(TabIndex = 0은 이 버튼이 focus를 받는 첫 번째 컨트롤로 만든다)
만든 button을 아랫쪽 하단 가운데에 배치
4개의 NumericUpDown을 위에서 부터 아래쪽으로 순서대로
TabIndex를 1~4로 설정
sum - 1
difference - 2
product - 3
quotient - 4
만든 앱을 실행해서 Tab 키를 눌러서
TabIndex가 위에 설정한 순서대로 작동하는지 확인
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace MathQuiz
{
public partial class Form1 : Form
{
Random randomizer = new Random();
int addend1;
int addend2;
int minuend;
int subtrahend;
int multiplicand;
int multiplier;
int dividend;
int divisor;
public void StartTheQuiz()
{
//더하기
// 0 ~ 50의 난수 반환
addend1 = randomizer.Next(51);
addend2 = randomizer.Next(51);
plusLeftLabel.Text = addend1.ToString();
plusRightLabel.Text = addend2.ToString();
sum.Value = 0;
//빼기
minuend = randomizer.Next(1, 101);
subtrahend = randomizer.Next(1, minuend);
minusLeftLabel.Text = minuend.ToString();
minusRightLabel.Text = subtrahend.ToString();
difference.Value = 0;
//곱하기
multiplicand = randomizer.Next(2, 11);
multiplier = randomizer.Next(2, 11);
timesLeftLabel.Text = multiplicand.ToString();
timesRightLabel.Text = multiplier.ToString();
product.Value = 0;
//나누기
divisor = randomizer.Next(2, 11);
int temporaryQuotient = randomizer.Next(2, 11);
dividend = divisor * temporaryQuotient;
dividedLeftLabel.Text = dividend.ToString();
dividedRightLabel.Text = divisor.ToString();
quotient.Value = 0;
}
public Form1()
{
InitializeComponent();
}
private void startBtn_Click(object sender, EventArgs e)
{
StartTheQuiz();
startBtn.Enabled = false;
}
}
}
다음과 같이 코드 입력
앱을 실행하고
Start the quiz 버튼을 누르면
난수 수학 문제가 나타나는지 확인
도구상자 - 구성요소 - Timer 를 Form에 추가
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace MathQuiz
{
public partial class Form1 : Form
{
Random randomizer = new Random();
int addend1;
int addend2;
int minuend;
int subtrahend;
int multiplicand;
int multiplier;
int dividend;
int divisor;
int timeLeft;
private bool CheckTheAnswer()
{
if ((addend1 + addend2 == sum.Value)
&& (minuend - subtrahend == difference.Value)
&& (multiplicand * multiplier == product.Value)
&& (dividend / divisor == quotient.Value))
return true;
else
return false;
}
public void StartTheQuiz()
{
//더하기
// 0 ~ 50의 난수 반환
addend1 = randomizer.Next(51);
addend2 = randomizer.Next(51);
plusLeftLabel.Text = addend1.ToString();
plusRightLabel.Text = addend2.ToString();
sum.Value = 0;
//빼기
minuend = randomizer.Next(1, 101);
subtrahend = randomizer.Next(1, minuend);
minusLeftLabel.Text = minuend.ToString();
minusRightLabel.Text = subtrahend.ToString();
difference.Value = 0;
//곱하기
multiplicand = randomizer.Next(2, 11);
multiplier = randomizer.Next(2, 11);
timesLeftLabel.Text = multiplicand.ToString();
timesRightLabel.Text = multiplier.ToString();
product.Value = 0;
//나누기
divisor = randomizer.Next(2, 11);
int temporaryQuotient = randomizer.Next(2, 11);
dividend = divisor * temporaryQuotient;
dividedLeftLabel.Text = dividend.ToString();
dividedRightLabel.Text = divisor.ToString();
quotient.Value = 0;
//시작 타이머 설정
timeLeft = 30;
timeLabel.Text = "30 seconds";
timer1.Start();
}
public Form1()
{
InitializeComponent();
}
private void startBtn_Click(object sender, EventArgs e)
{
StartTheQuiz();
startBtn.Enabled = false;
}
private void timer1_Tick(object sender, EventArgs e)
{
if (CheckTheAnswer())
{
// 모든 정답을 맞췄을때
timer1.Stop();
MessageBox.Show("You got all the answers right!",
"Congratulations!");
startBtn.Enabled = true;
}
else if (timeLeft > 0)
{
// 시간이 0초보다 많을때
timeLeft = timeLeft - 1;
timeLabel.Text = timeLeft + " seconds";
}
else
{
// 정답을 못 맟줬고, 시간이 모두 지났을때
// 정답이 공개된다.
timer1.Stop();
timeLabel.Text = "Time's up!";
MessageBox.Show("You didn't finish in time.", "Sorry!");
sum.Value = addend1 + addend2;
difference.Value = minuend - subtrahend;
product.Value = multiplicand * multiplier;
quotient.Value = dividend / divisor;
startBtn.Enabled = true;
}
}
}
}
다음과 같이 코드 입력
앱을 실행하고
버튼을 누르면 타이머가 카운트 다운을 시작하는지 확인
카운트 다운이 끝나면 메세지 박스가 나오고
박스를 종료하면 정답이 표시된다.
NumericUpDown을 선택하고 속성창에서 이밴트 탭(번개)을 클릭
Enter - answer_Enter를 입력 (팝업박스에서 선택 x, 직접 입력)하고 엔터를 누른다.
그럼 코드 편집기(Form1.cs)가 나오고, 컨트롤에서 만든 answer_enter 메서드가 추가된다.
private void answer_enter(object sender, EventArgs e)
{
// NumericUpDown의 모든 답변을 선택(answerBox)
NumericUpDown answerBox = sender as NumericUpDown;
// 모든 답변이 null이 아니라면
// Tab 키로 포커스를 이동할 때 해당 NumericUpDown에 적혀있는 값이 모두 선택이 된다.
if (answerBox != null)
{
int lengthOfAnswer = answerBox.Value.ToString().Length;
answerBox.Select(0, lengthOfAnswer);
}
}
다음과 같이 코드 작성
기존의 Tab으로 Focus 이동시
코드 작성 후, Tab으로 이동 시
적힌 값들이 모두 선택 되어 있는 것을 확인
최종 실행 이미지
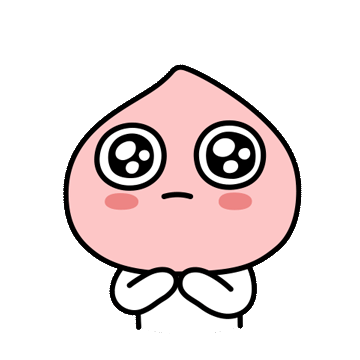
'Window Forms 공부' 카테고리의 다른 글
[Windows Forms] 사진 뷰어 앱 만들어보기 (0) | 2024.05.29 |
---|---|
[Windows Forms] 계산기 만들어보기 (0) | 2024.05.27 |
[Windows Forms] String 버튼 만들기 (0) | 2024.05.25 |
Windows Forms App과 Windows Forms App (.NET Framwork)의 차이점은 무엇일까? (0) | 2024.05.25 |