using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BulletController : MonoBehaviour
{
[SerializeField] private float force = 1000f;
[Range(0, 1)][SerializeField] private float reflectionBias = 0.5f; // 반사각도 조정
private Rigidbody rBody;
void Start()
{
rBody = this.GetComponent<Rigidbody>();
rBody.AddRelativeForce(Vector3.forward * this.force);
}
void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.CompareTag("Wall"))
{
Vector3 reflectDirection = Vector3.Reflect(rBody.velocity.normalized, collision.contacts[0].normal);
reflectDirection = Vector3.Lerp(reflectDirection, collision.contacts[0].normal, reflectionBias);
rBody.velocity = reflectDirection * rBody.velocity.magnitude;
// 반사된 방향으로 총알 회전
transform.rotation = Quaternion.LookRotation(rBody.velocity);
// 총알의 회전 속도를 0으로 설정
rBody.angularVelocity = Vector3.zero;
}
}
}
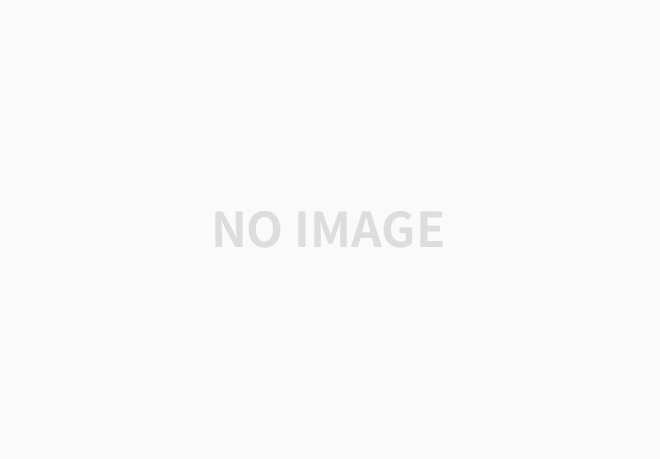
반응형
'KDT > 유니티 심화' 카테고리의 다른 글
[SpaceShooter] 가장 가까운 적에게 총 발사하기 (0) | 2023.08.22 |
---|---|
[SpaceShooter] 가까운 오브젝트 장착하기 (OverlapSphereNonAlloc) (0) | 2023.08.21 |
[SpaceShooter] 벽에 닿았을 때 캐릭터 회전 시키기 (2) (0) | 2023.08.21 |
[SpaceShooter] 벽에 닿았을때 캐릭터 회전시키기 (1) (0) | 2023.08.21 |
[궁수의 전설 따라해보기] Legend of Circle (0) | 2023.08.21 |