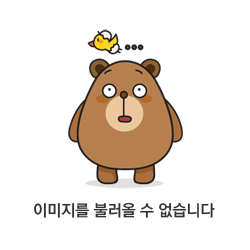
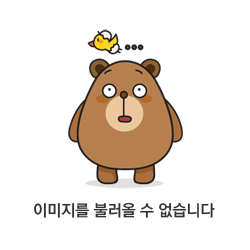
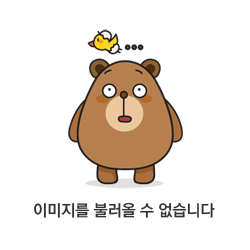
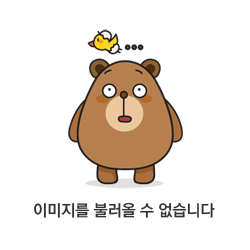
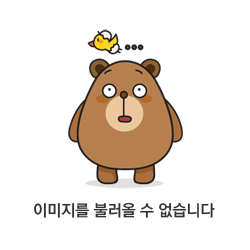
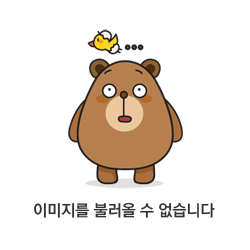
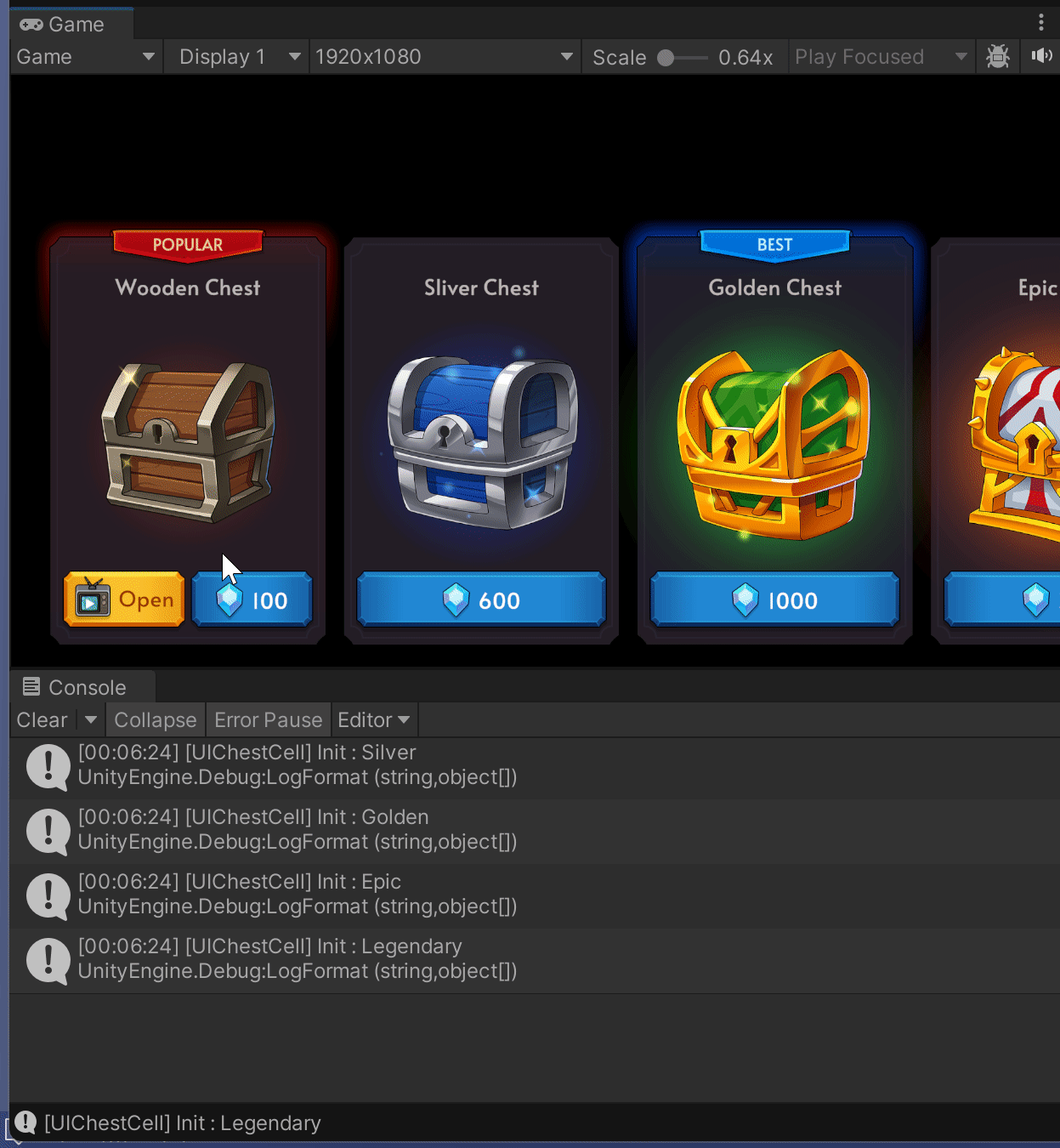
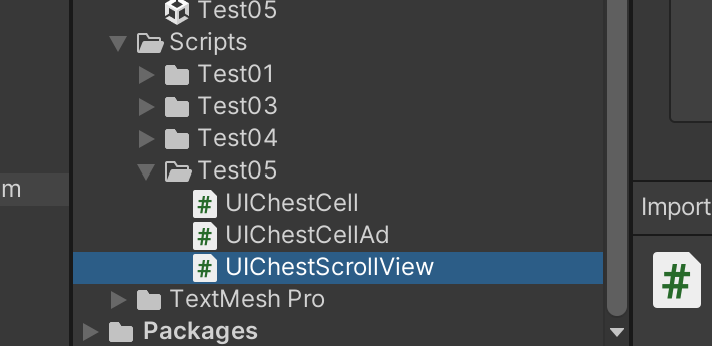
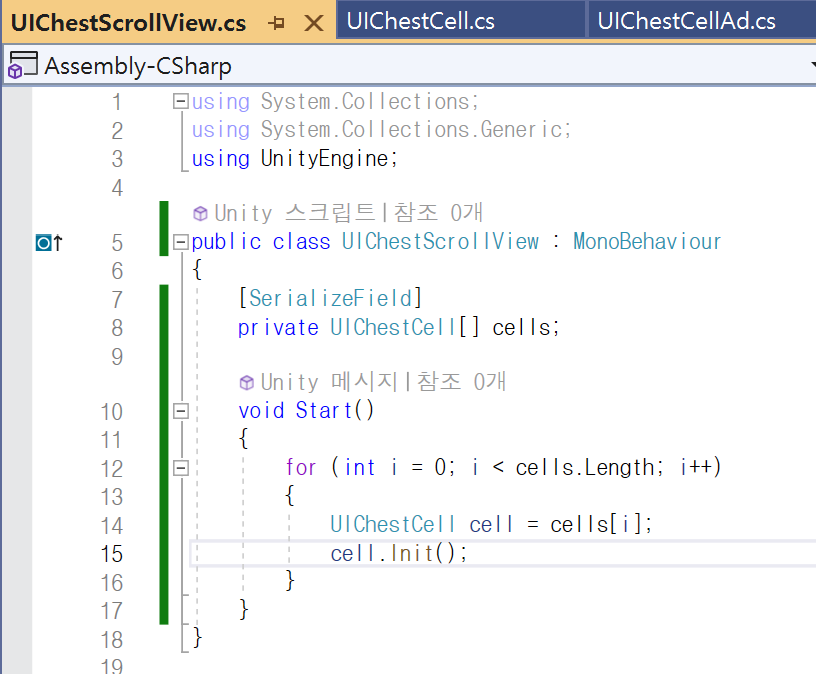
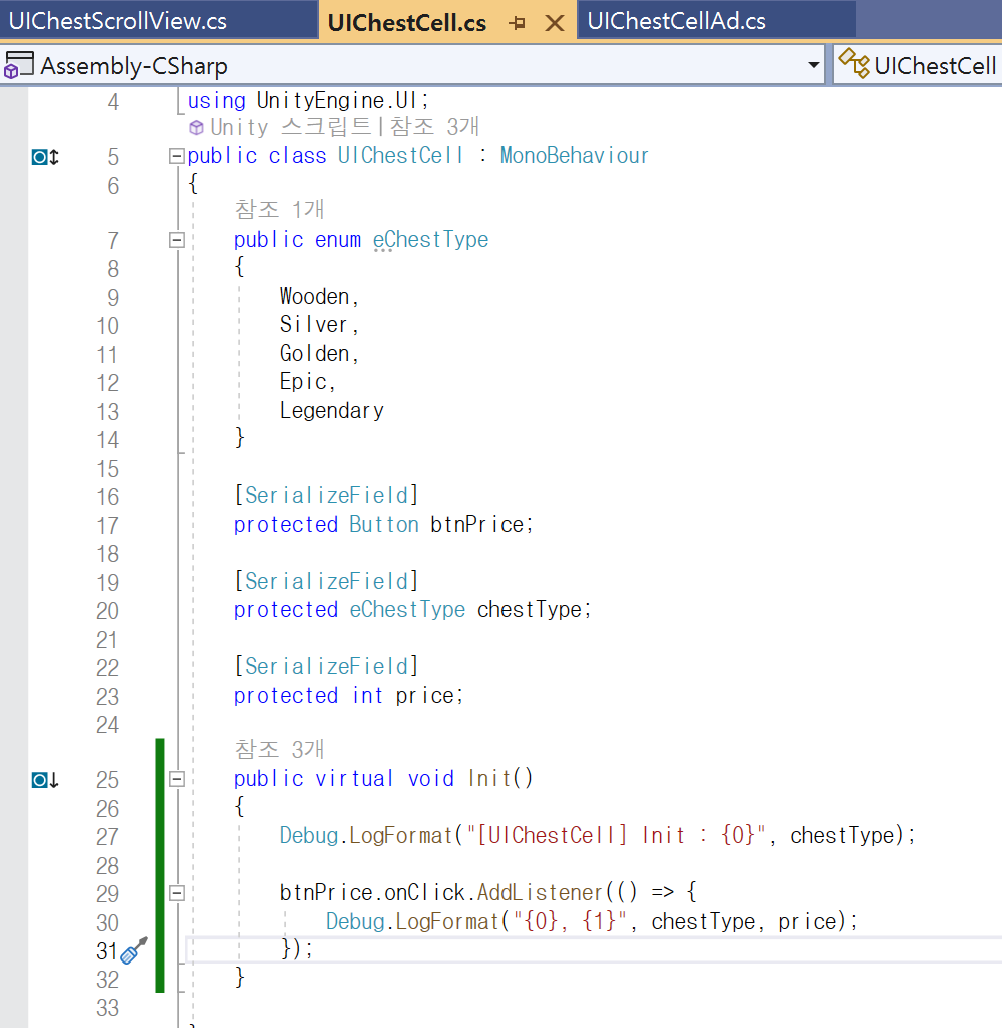
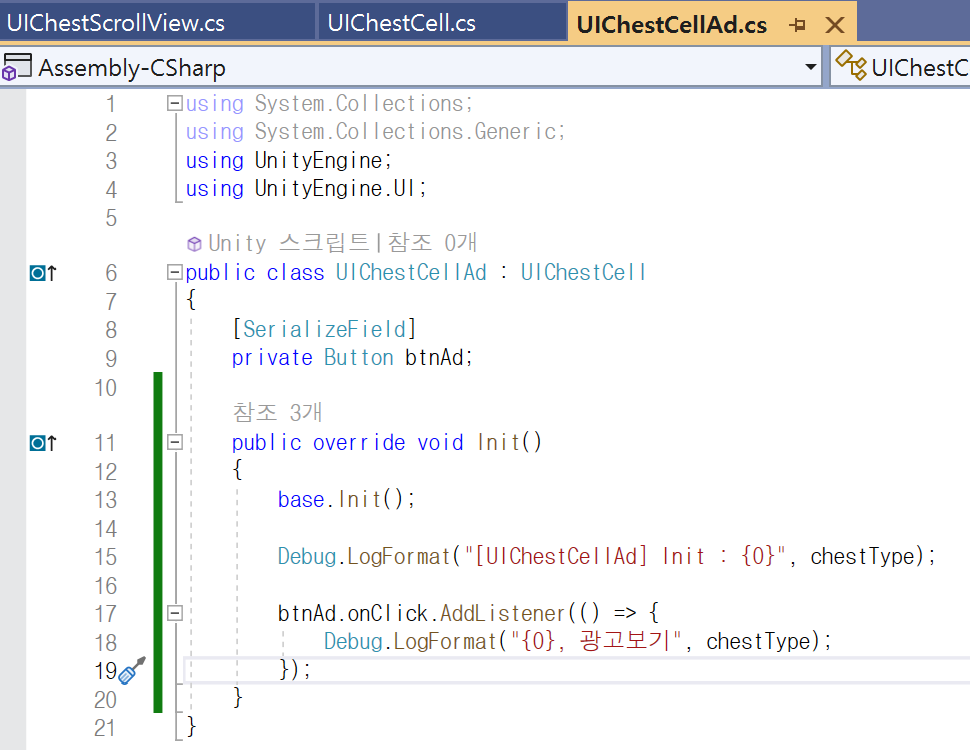
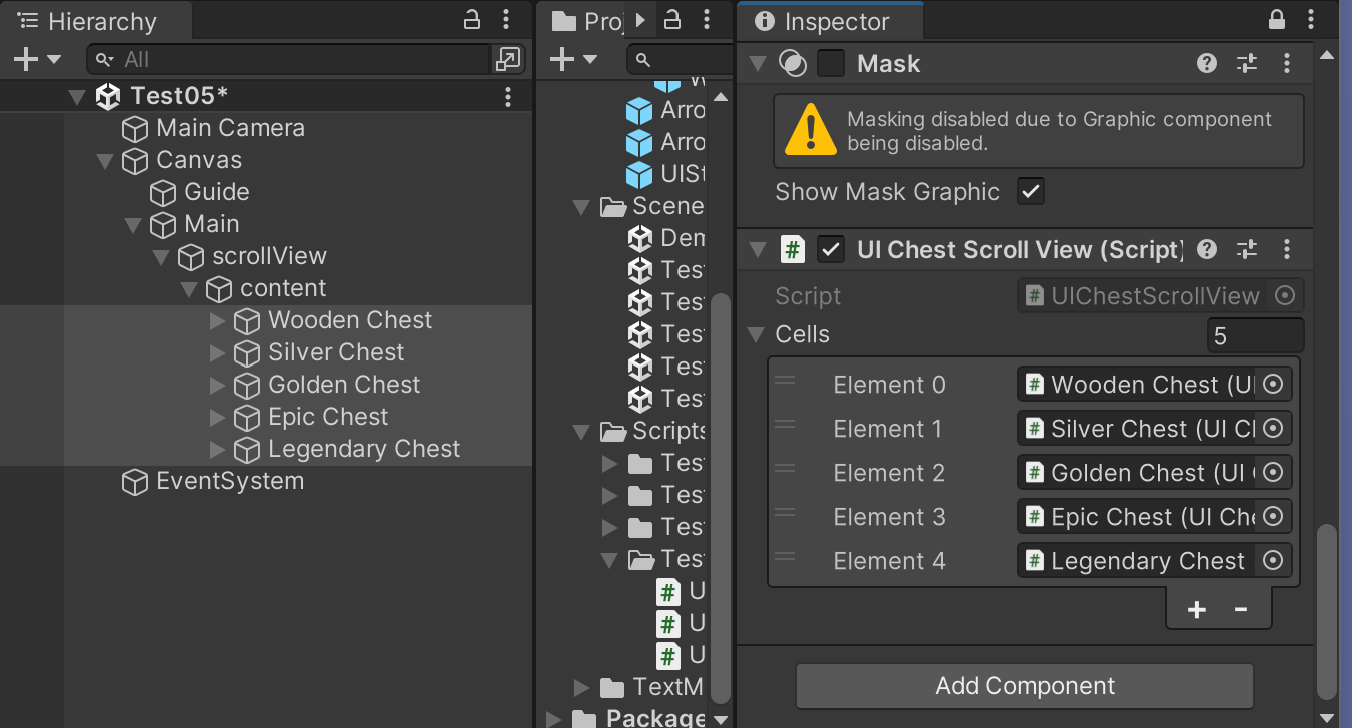
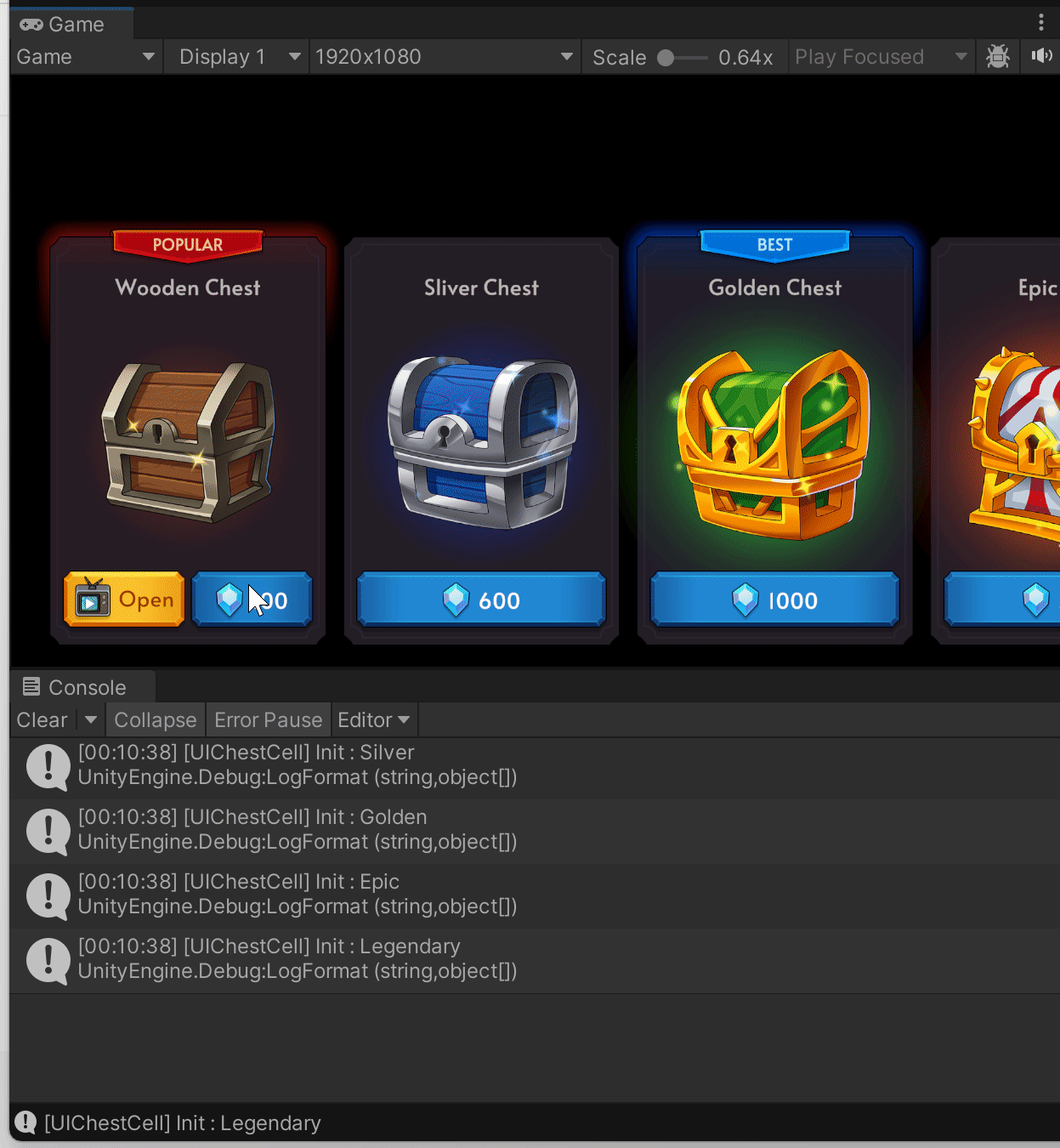
+++
람다와 대리자를 통해 이벤트 처리와 콜백을 구현해보기
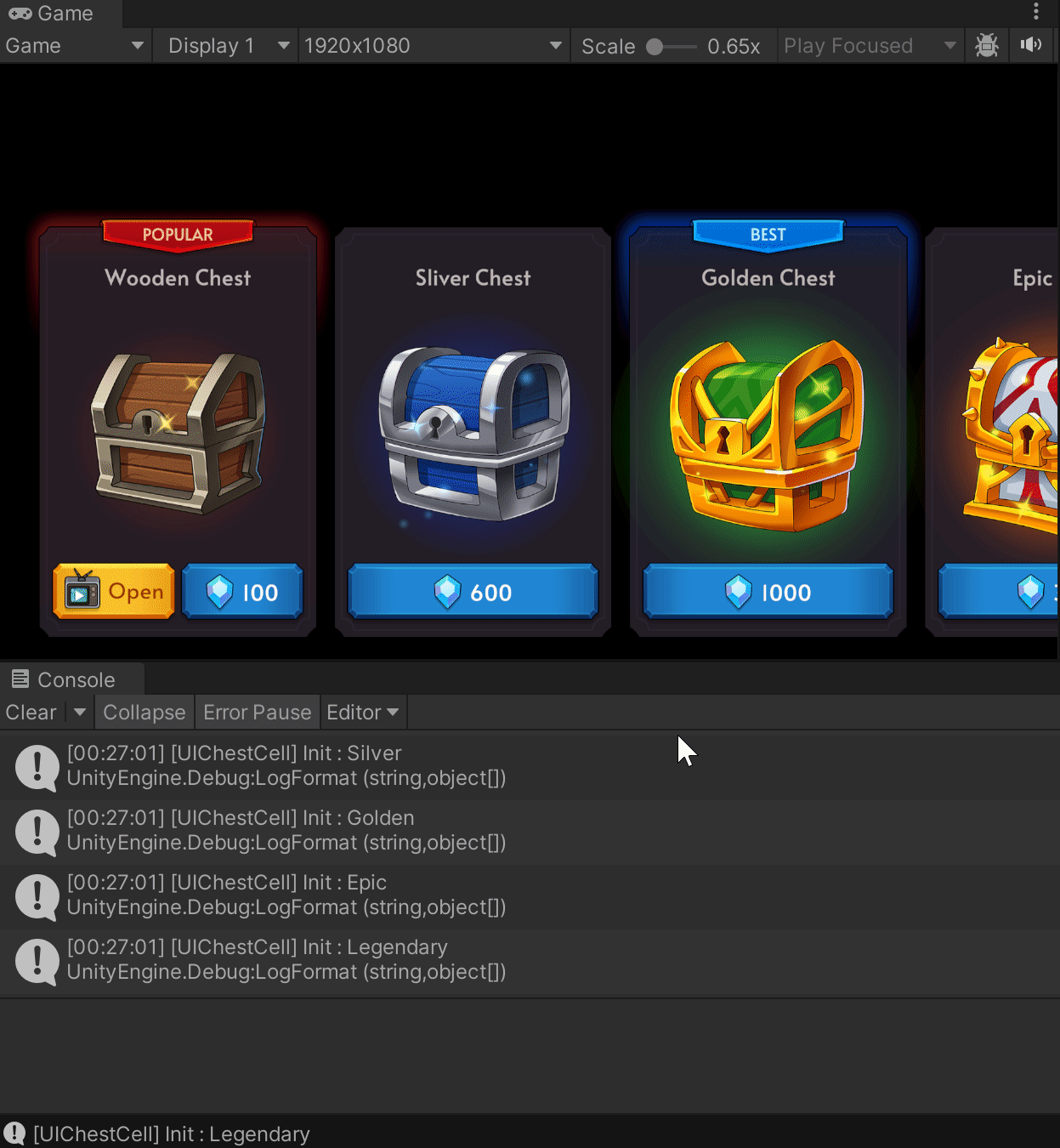
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class UIChestScrollView : MonoBehaviour
{
[SerializeField]
private UIChestCell[] cells;
void Start()
{
for (int i = 0; i < cells.Length; i++)
{
UIChestCell cell = cells[i];
cell.onClickPrice = () => {
Debug.LogFormat("<color=red>{0}, {1}</color>", cell.ChestType, cell.Price);
};
var chestType = (UIChestCell.eChestType)i;
if (chestType == UIChestCell.eChestType.Wooden)
{
var cellAd = (UIChestCellAd)cell;
cellAd.onClickAd = () =>
{
Debug.LogFormat("<color=blue>{0}, 광고보기</color>", cell.ChestType);
};
}
cell.Init();
}
}
}
14. UIChestScrollView 스크립트
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class UIChestCell : MonoBehaviour
{
public enum eChestType
{
Wooden,
Silver,
Golden,
Epic,
Legendary
}
[SerializeField]
protected Button btnPrice;
[SerializeField]
protected eChestType chestType;
[SerializeField]
protected int price;
public eChestType ChestType
{
get
{
return this.chestType;
}
}
public int Price => price;
//public int Price -> 동일한 기능을 하는 코드
//{
// get { return this.price; }
//}
public System.Action onClickPrice;
public virtual void Init()
{
Debug.LogFormat("[UIChestCell] Init : {0}", chestType);
this.btnPrice.onClick.AddListener(() => {
Debug.LogFormat("{0}, {1}", chestType, price);
onClickPrice();
});
}
}
15. UIChestCell 스크립트
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class UIChestCellAd : UIChestCell
{
[SerializeField]
private Button btnAd;
public System.Action onClickAd;
public override void Init()
{
base.Init();
Debug.LogFormat("[UIChestCellAd] Init : {0}", chestType);
btnAd.onClick.AddListener(() => {
Debug.LogFormat("{0}, 광고보기", chestType);
onClickAd();
});
}
}
16. UIChestCellAd 스크립트
반응형
'KDT > 유니티 심화' 카테고리의 다른 글
LearnUGUI (Gold Packs - 데이터 테이블 만들고 연동하기) - 1 (0) | 2023.09.11 |
---|---|
LearnUGUI연습 (8. ShopChest 데이터 연동 하기) (0) | 2023.09.10 |
LearnUGUI 연습 (6. ShopChest 정적 스크롤뷰 만들기) (0) | 2023.09.09 |
LearnUGUI 연습 (5. UIPageStage 단계 별 적용하기) (0) | 2023.09.08 |
LearnUGUI 연습 (4. 데이터 연동을 통한 Stage 만들기) (0) | 2023.09.07 |