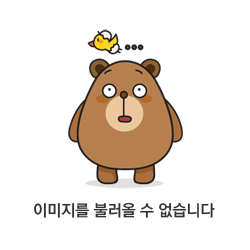
using System.Collections;
using System.Collections.Generic;
using Unity.VisualScripting;
using UnityEditor;
using UnityEngine;
using UnityEngine.UI;
public class ArrowController : MonoBehaviour
{
[SerializeField] private float speed = 1f;
[SerializeField] private float radius = 1;
private CatGameDIrector catGameDIrector;
private GameObject playerGo;
[SerializeField] private float damage = 0.5f;
void Start()
{
this.playerGo = GameObject.Find("player");//이름으로 컴포넌트를 찾는다.
this.catGameDIrector = GameObject.FindObjectOfType<CatGameDIrector>();//타입으로 컴포넌트를 찾는다.
}
void Update()
{
if(catGameDIrector.healthGage.fillAmount <= 0)
{
Destroy(this.gameObject);
}
this.gameObject.transform.Translate(0, -0.1f, 0 * speed * Time.deltaTime);
if(this.gameObject.transform.position.y < -2.93f)
{
catGameDIrector.RemoveArrowFromList(this.gameObject); // 리스트에서 화살 제거
Destroy(this.gameObject);
}
Vector2 p1 = this.transform.position;
Vector2 p2 = this.playerGo.transform.position;
Vector2 dir = p1 - p2;
float distance = dir.magnitude;
float r1 = this.radius;
float r2 = this.playerGo.GetComponent<PlayerController>().radius;
float sumRadius = r1 + r2;
if(distance < sumRadius) // Arrow와 Player가 충돌시
{
this.catGameDIrector.DecreaseHp(damage);
Destroy (this.gameObject); // 씬에서 제거
}
}
private void OnDrawGizmos()
{
Gizmos.color = Color.yellow;
Gizmos.DrawWireSphere(this.transform.position, this.radius);
}
}
2. ArrowController
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class CatGameDIrector : MonoBehaviour
{
[SerializeField] private GameObject player;
[SerializeField] private GameObject arrowPrefab;
[SerializeField] private float recycleTime = 3;
[SerializeField] private Text gameoverText;
private float delta;
public Image healthGage; // Inspector에서 할당할 HP 게이지 Image
private List<GameObject> arrows = new List<GameObject>(); // 화살 추적을 위한 리스트
void Start()
{
gameoverText.gameObject.SetActive(false);
}
void Update()
{
delta += Time.deltaTime; // 이전 프레임과 현재 프레임 사이 시간
if (healthGage.fillAmount <= 0)
{
gameoverText.gameObject.SetActive(true);
foreach (GameObject arrow in arrows)
{
Destroy(arrow); // 모든 화살 삭제
}
arrows.Clear(); // 리스트 비우기
}
else
{
if (delta > recycleTime) // recycleTime 보다 크다면
{
GameObject go = Instantiate(arrowPrefab);
float randX = Random.Range(-8f, 9f); // -8 ~ 8
go.transform.position = new Vector3(randX, go.transform.position.y, go.transform.position.z);
arrows.Add(go); // 리스트에 화살 추가
delta = 0f;
}
}
}
public void LeftButton()
{
if(healthGage.fillAmount > 0)
{
player.gameObject.transform.Translate(-2, 0, 0);
float clampedX = Mathf.Clamp(player.transform.position.x, -8f, 8f);
player.transform.position = new Vector3(clampedX, player.transform.position.y, player.transform.position.z);
}
}
public void RightButton()
{
if (healthGage.fillAmount > 0)
{
player.gameObject.transform.Translate(2, 0, 0);
float clampedX = Mathf.Clamp(player.transform.position.x, -8f, 8f);
player.transform.position = new Vector3(clampedX, player.transform.position.y, player.transform.position.z);
}
}
public void DecreaseHp(float damage)
{
if(healthGage.fillAmount <= 0)
{
}
else
{
this.healthGage.fillAmount -= damage;
}
}
public void RemoveArrowFromList(GameObject arrow)
{
arrows.Remove(arrow);
}
}
3.CatGameDIrector
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class PlayerController : MonoBehaviour
{
[SerializeField] private CatGameDIrector CatGameDIrector;
public float radius = 1;
void Start()
{
}
void Update()
{
if(CatGameDIrector.healthGage.fillAmount > 0f)
{
if (Input.GetKeyDown(KeyCode.LeftArrow))
{
this.transform.Translate(-2, 0, 0);
}
if (Input.GetKeyDown(KeyCode.RightArrow))
{
this.transform.Translate(2, 0, 0);
}
float clampedX = Mathf.Clamp(this.transform.position.x, -8f, 8f);
this.transform.position = new Vector3(clampedX, this.transform.position.y, this.transform.position.z);
}
}
private void OnDrawGizmos()
{
Gizmos.color = Color.yellow;
Gizmos.DrawWireSphere(this.transform.position, this.radius);
}
}
4. PlayerController
반응형
'산대특 > 게임 클라이언트 프로그래밍' 카테고리의 다른 글
Update와 Coroutine (0) | 2024.02.02 |
---|---|
[ClimbCloud] 캐릭터를 AddForce로 이동 및 좌우 전환 만들어보기 (0) | 2024.01.31 |
[Shuriken Swipe] 수리검 이동 및 회전 + 도착 지점 계산하기 (0) | 2024.01.27 |
[Chapter4] Swipe Car 만들기 (0) | 2024.01.26 |
[Chapter3] 룰렛 회전시키기 (0) | 2024.01.26 |