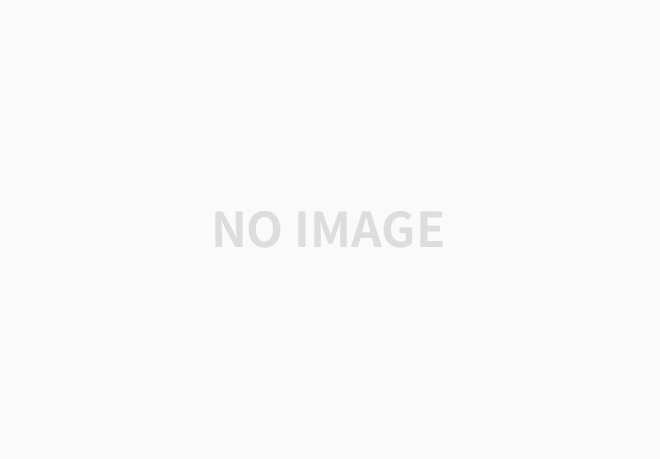
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ParticlePoolManager : MonoBehaviour
{
public static ParticlePoolManager instance;
private List<GameObject> particlePool = new List<GameObject>();
[SerializeField]
private int maxParticles = 10;
[SerializeField]
private GameObject particlePrefab;
private void Awake()
{
instance = this;
DontDestroyOnLoad(this.gameObject);
}
void Start()
{
for (int i = 0; i < this.maxParticles; i++)
{
GameObject particleGo = Instantiate(this.particlePrefab);
particleGo.SetActive(false);
particleGo.transform.SetParent(this.transform);
particlePool.Add(particleGo);
}
}
public GameObject GetParticle()
{
foreach (GameObject particleGo in particlePool)
{
if (!particleGo.activeSelf)
{
particleGo.transform.SetParent(null);
return particleGo;
}
}
return null;
}
public void ReleaseParticle(GameObject particleGo, float delay)
{
StartCoroutine(ReleaseAfterDelay(particleGo, delay));
}
private IEnumerator ReleaseAfterDelay(GameObject particleGo, float delay)
{
yield return new WaitForSeconds(delay);
particleGo.SetActive(false);
particleGo.transform.SetParent(this.transform);
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TestBulletPoolManager : MonoBehaviour
{
public static TestBulletPoolManager instance;
private List<GameObject> pool = new List<GameObject>();
[SerializeField]
private int maxBullets = 10;
[SerializeField]
private GameObject testBulletPrefab;
private void Awake()
{
instance = this;
DontDestroyOnLoad(this.gameObject);
}
void Start()
{
for (int i = 0; i < this.maxBullets; i++)
{
GameObject bulletGo = Instantiate(this.testBulletPrefab);
bulletGo.SetActive(false);
bulletGo.transform.SetParent(this.transform);
this.pool.Add(bulletGo);
}
}
public GameObject GetBullet()
{
foreach (GameObject bulletGo in this.pool)
{
if (bulletGo.activeSelf == false)
{
bulletGo.transform.SetParent(null);
return bulletGo;
}
}
return null;
}
//풀에 반환
public void Release(GameObject bulletGo)
{
bulletGo.SetActive(false);
bulletGo.transform.SetParent(this.transform);
}
}
using UnityEngine;
using UnityEngine.UI;
public class TestObjectPoolingMain : MonoBehaviour
{
[SerializeField]
private Button btn;
[SerializeField]
private TestBulletPoolManager bulletPool;
private Vector3 shootDirection = Vector3.forward;
private void Start()
{
this.btn.onClick.AddListener(() => {
GameObject bulletGo = bulletPool.GetBullet();
if (bulletGo != null)
{
bulletGo.transform.position = Vector3.zero;
bulletGo.transform.rotation = Quaternion.LookRotation(shootDirection); // 총알의 회전을 발사 방향으로 설정
TestBullet1 bulletScript = bulletGo.GetComponent<TestBullet1>();
bulletScript.SetDirection(shootDirection);
bulletGo.SetActive(true);
}
// Rotate the shoot direction by 90 degrees around the y axis.
shootDirection = Quaternion.Euler(0, 90, 0) * shootDirection;
});
}
}
using UnityEngine;
public class TestBullet1 : MonoBehaviour
{
[SerializeField]
private float speed = 1f;
private Vector3 direction;
public void SetDirection(Vector3 dir)
{
direction = dir;
}
void Update()
{
this.transform.Translate(direction * this.speed * Time.deltaTime, Space.World);
}
private void OnCollisionEnter(Collision collision)
{
if (collision.collider.CompareTag("Wall"))
{
Debug.Log("Bullet Hit Wall");
// 파티클 생성 및 활성화
GameObject particleGo = ParticlePoolManager.instance.GetParticle();
if (particleGo != null)
{
ContactPoint cp = collision.GetContact(0); //충돌 지점의 정보 추출
Quaternion rot = Quaternion.LookRotation(-cp.normal); //충돌한 총알의 법선 벡터를 쿼터니언 타입으로 변환
particleGo.transform.rotation = rot;
particleGo.transform.position = cp.point;
particleGo.transform.SetParent(ParticlePoolManager.instance.transform);
particleGo.SetActive(true);
ParticlePoolManager.instance.ReleaseParticle(particleGo, 1f);
particleGo.transform.position = collision.contacts[0].point;
particleGo.SetActive(true);
ParticlePoolManager.instance.ReleaseParticle(particleGo, 3f); // 3초 후에 파티클 반환
}
TestBulletPoolManager.instance.Release(this.gameObject);
}
}
}
반응형
'KDT > 유니티 심화' 카테고리의 다른 글
[SpaceShooter] 라이트 프로브 연습 (0) | 2023.08.31 |
---|---|
[SpaceShooter] 라이트 매핑 연습 (0) | 2023.08.31 |
[SpaceShooter] Player 피격시 체력 바 감소(UI) 구현 (0) | 2023.08.25 |
[SpaceShooter] Player와 Monster 사망 시 동작(애니메이션) 구현 (0) | 2023.08.24 |
[SpaceShooter] Monster 공격과 피격 구현 (0) | 2023.08.24 |