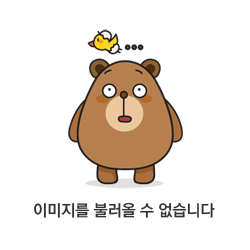
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SpaceShipController : MonoBehaviour
{
int speed = 5; // 스피드
[SerializeField] GameObject bulletPrefab;
[SerializeField] Transform shootPosition;
private Animator anim;
private enum State
{
Idle, Left, Right
}
private void Start()
{
anim = GetComponent<Animator>();
StartCoroutine("CoMove");
}
void Update()
{
// 스페이스바를 누를 때 CoShooting 코루틴을 시작
if (Input.GetKeyDown(KeyCode.Space))
{
StartCoroutine("CoShooting");
}
}
IEnumerator CoMove()
{
while (true)
{
float h = Input.GetAxisRaw("Horizontal");
float v = Input.GetAxisRaw("Vertical");
if (h > 0)
{
anim.SetInteger("State", 2);
}
else if( h < 0)
{
anim.SetInteger("State", 1);
}
else
{
anim.SetInteger("State",0);
}
Vector2 inputDirection = new Vector2(h, v).normalized; // 입력 방향 정규화
float xMove = inputDirection.x * speed * Time.deltaTime;
float yMove = inputDirection.y * speed * Time.deltaTime;
this.transform.Translate(new Vector2(xMove, yMove));
yield return null;
}
}
IEnumerator CoShooting()
{
// 총알 프리팹을 shootPosition에서 생성하고, 위쪽으로 발사
GameObject bullet = Instantiate(bulletPrefab, shootPosition.position, Quaternion.identity);
Rigidbody2D rb = bullet.GetComponent<Rigidbody2D>();
if (rb != null)
{
rb.velocity = Vector2.up * speed;
}
yield return null;
}
IEnumerator CoAnimation()
{
yield return null;
}
}
2. SpaceShipController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyController : MonoBehaviour
{
[SerializeField] private int enemyHP = 3;
private Animator anim;
void Start()
{
anim = GetComponent<Animator>();
}
// Update is called once per frame
void Update()
{
}
private void OnTriggerEnter2D(Collider2D collision)
{
if (collision.gameObject.CompareTag("Bullet"))
{
this.enemyHP -= 1;
anim.SetInteger("State", 1);
Destroy(collision.gameObject);
}
if (this.enemyHP <= 0)
{
Destroy(this.gameObject);
}
}
}
3. EnemyController.cs
using UnityEngine;
public class ReturnIdle : MonoBehaviour
{
private Animator anim;
void Start()
{
anim = GetComponent<Animator>();
}
public void ResetToIdle()
{
anim.SetInteger("State", 0); // Idle 상태로 설정
}
}
4. ReturnIdle.cs (애니메이션 이벤트)
배경 스크롤링을 진행 하다가
시간이 너무 늦어져서
일단 여기까지 올립니다.
반응형
'산대특 > 게임 클라이언트 프로그래밍' 카테고리의 다른 글
Unity에서 사용되는 시간 관련 메서드들과 로컬 시간및 UTC (0) | 2024.03.26 |
---|---|
[AppleCatch] AppleCatch 만들기 (0) | 2024.02.05 |
[PirateBomb] 캐릭터의 이동과 공격, 적의 피격과 사망 (0) | 2024.02.05 |
[SpaceShooter2D] 비행기[Player] GetAxis로 이동하기 + 정규화(Normalize) (0) | 2024.02.02 |
[Bamsongi] 화면 클릭해서 밤송이 던지기 + 파티클 (0) | 2024.02.02 |