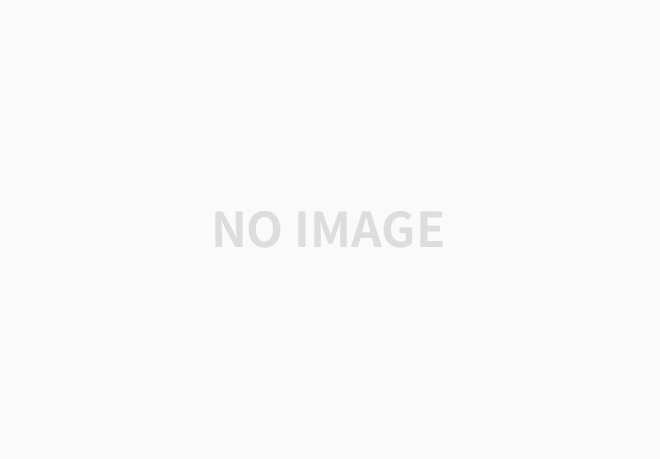
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MouseClick : MonoBehaviour
{
[SerializeField] private GameObject basket;
void Update()
{
if (Input.GetMouseButtonDown(0)) // 마우스 왼쪽 버튼이 클릭되었는지 확인
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition); // 마우스 위치에서 레이 생성
RaycastHit hit; // 충돌 정보를 저장할 객체
if (Physics.Raycast(ray, out hit)) // 레이캐스트가 어떤 객체와 충돌했는지 확인
{
// 충돌한 지점의 x와 z 축 위치를 반올림
float roundedX = Mathf.Round(hit.point.x);
float roundedZ = Mathf.Round(hit.point.z);
// basket의 위치를 충돌 지점의 반올림된 x, z 위치로 설정 (y축은 0)
basket.transform.position = new Vector3(roundedX, 0f, roundedZ);
Debug.LogFormat("바구니 이동 좌표: {0}", basket.transform.position);
}
else
{
// 레이가 아무것도 충돌하지 않았을 경우
Debug.Log("레이가 충돌되지 않음");
}
}
}
}
1. MouseClick.cs (바구니 이동 스크립트)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ItemController2 : MonoBehaviour
{
[SerializeField] private float moveSpeed = 1f;
void Start()
{
}
// Update is called once per frame
void Update()
{
this.transform.Translate(Vector3.down * moveSpeed*Time.deltaTime);
if(this.transform.position.y <= 0)
{
Destroy(this.gameObject);
}
}
}
2. ItemController2 (apple,bomb 제거 스크립트)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class ItemGenerator : MonoBehaviour
{
[SerializeField] private GameObject applePrefab;
[SerializeField] private GameObject bombPrefab;
[SerializeField] private Text TimeText;
[SerializeField]private float remainingTime = 60.0f; // 남은 시간을 60초로 초기화
void Start()
{
StartCoroutine(PrefabGenerator());
}
void Update()
{
if (remainingTime > 0)
{
// 매 프레임마다 시간 감소
remainingTime -= Time.deltaTime;
// 시간을 텍스트 형식으로 업데이트 (소수점 두 자리까지 표시)
TimeText.text = remainingTime.ToString("00.00");
}
else
{
// 시간이 0에 도달하면 00.00으로 고정
TimeText.text = "00.00";
SceneManager.LoadScene(1);
// 추가적인 처리가 필요한 경우 여기에 코드 추가 (예: 게임 오버 처리)
}
}
IEnumerator PrefabGenerator()
{
while (remainingTime > 0) // 남은 시간이 있을 때만 객체 생성 계속
{
int randX = Random.Range(-1, 2); // -1, 0, 또는 1 사이에서 랜덤 값을 선택
int randZ = Random.Range(-1, 2); // -1, 0, 또는 1 사이에서 랜덤 값을 선택
int randGO = Random.Range(0, 2); // 0 또는 1을 랜덤으로 선택
if (randGO == 0)
{
// apple 생성
Instantiate(applePrefab, new Vector3(randX, 3, randZ), Quaternion.identity);
}
else
{
// bomb 생성
Instantiate(bombPrefab, new Vector3(randX, 3, randZ), Quaternion.identity);
}
yield return new WaitForSeconds(2); // 2초 동안 대기
}
}
}
3. ItemGenerator.cs ( apple,bomb 생성 스크립트)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RealDirector : MonoBehaviour
{
public int sumApple = 0;
public int sumBomb = 0;
public int sumScore = 0;
void Start()
{
DontDestroyOnLoad(gameObject); // 현재 게임 오브젝트를 씬 전환 시 파괴되지 않도록 설정
}
// Update is called once per frame
void Update()
{
}
}
4. RealDirector.cs (다음 씬으로 데이터 넘겨주는 스크립트)
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class BasketController : MonoBehaviour
{
[SerializeField] private AudioClip appleSfx;
[SerializeField] private AudioClip bombSfx;
[SerializeField] private Text ScoreText;
[SerializeField ]private RealDirector realDirector = new RealDirector();
private float score = 0f;
private AudioSource AudioSource;
// Start is called before the first frame update
void Start()
{
this.AudioSource = this.GetComponent<AudioSource>();
ScoreText.text = Convert.ToString((int)Math.Floor(this.score));
realDirector.sumScore = 0;
}
// Update is called once per frame
void Update()
{
realDirector.sumScore = (int)Math.Floor(this.score);
}
private void OnTriggerEnter(Collider other)
{
Debug.LogFormat("잡았다! => : {0}",other.gameObject.tag);
if(other.gameObject.tag == "Apple")
{
Debug.Log("득점");
score += 100;
ScoreText.text = Convert.ToString(score);
realDirector.sumApple += 1;
this.AudioSource.PlayOneShot(this.appleSfx);
}
else if(other.gameObject.tag == "Bomb")
{
Debug.Log("감점");
score = score *= 0.5f;
ScoreText.text = Convert.ToString(score);
realDirector.sumBomb += 1;
this.AudioSource.PlayOneShot(this.bombSfx);
}
Destroy(other.gameObject);
}
}
5. BasketController.cs (바구니 스크립트)
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class Resultcontroller : MonoBehaviour
{
[SerializeField] Text appleText;
[SerializeField] Text bombText;
[SerializeField] Text scoreText;
[SerializeField] private Button reBtn;
// Start is called before the first frame update
void Start()
{
RealDirector realDirector = FindObjectOfType<RealDirector>();
appleText.text = "사과X" + Convert.ToString(realDirector.sumApple);
bombText.text = "폭탄X" + Convert.ToString(realDirector.sumBomb);
scoreText.text = "점수 : " + Convert.ToString(realDirector.sumScore);
reBtn.onClick.AddListener(() => {
Destroy(realDirector.gameObject);
SceneManager.LoadScene(0);
});
}
// Update is called once per frame
void Update()
{
}
}
6. Resultcontroller.cs (결과창 스크립트)
반응형
'산대특 > 게임 클라이언트 프로그래밍' 카테고리의 다른 글
Unity에서 사용되는 시간 관련 메서드들과 로컬 시간및 UTC (0) | 2024.03.26 |
---|---|
[ShootingGame] 이동, 발사, 충돌, 애니메이션(연출) 만들기 (0) | 2024.02.05 |
[PirateBomb] 캐릭터의 이동과 공격, 적의 피격과 사망 (0) | 2024.02.05 |
[SpaceShooter2D] 비행기[Player] GetAxis로 이동하기 + 정규화(Normalize) (0) | 2024.02.02 |
[Bamsongi] 화면 클릭해서 밤송이 던지기 + 파티클 (0) | 2024.02.02 |